
[ad_1]
SwiftUI’s structure primitives typically don’t present relative sizing choices, e.g. “make this view 50 % of the width of its container”. Let’s construct our personal!
Use case: chat bubbles
Contemplate this chat dialog view for instance of what I wish to construct. The chat bubbles at all times stay 80 % as vast as their container because the view is resized:
Constructing a proportional sizing modifier
1. The Format
We will construct our personal relative sizing modifier on prime of the Format
protocol. The structure multiplies its personal proposed measurement (which it receives from its mother or father view) with the given components for width and top. It then proposes this modified measurement to its solely subview. Right here’s the implementation (the complete code, together with the demo app, is on GitHub):
/// A customized structure that proposes a proportion of its
/// obtained proposed measurement to its subview.
///
/// - Precondition: should comprise precisely one subview.
fileprivate struct RelativeSizeLayout: Format {
var relativeWidth: Double
var relativeHeight: Double
func sizeThatFits(
proposal: ProposedViewSize,
subviews: Subviews,
cache: inout ()
) -> CGSize {
assert(subviews.depend == 1, "expects a single subview")
let resizedProposal = ProposedViewSize(
width: proposal.width.map { $0 * relativeWidth },
top: proposal.top.map { $0 * relativeHeight }
)
return subviews[0].sizeThatFits(resizedProposal)
}
func placeSubviews(
in bounds: CGRect,
proposal: ProposedViewSize,
subviews: Subviews,
cache: inout ()
) {
assert(subviews.depend == 1, "expects a single subview")
let resizedProposal = ProposedViewSize(
width: proposal.width.map { $0 * relativeWidth },
top: proposal.top.map { $0 * relativeHeight }
)
subviews[0].place(
at: CGPoint(x: bounds.midX, y: bounds.midY),
anchor: .heart,
proposal: resizedProposal
)
}
}
Notes:
-
I made the sort personal as a result of I wish to management how it may be used. That is vital for sustaining the belief that the structure solely ever has a single subview (which makes the maths a lot easier).
-
Proposed sizes in SwiftUI might be
nil
or infinity in both dimension. Our structure passes these particular values by unchanged (infinity occasions a proportion remains to be infinity). I’ll talk about beneath what implications this has for customers of the structure.
2. The View extension
Subsequent, we’ll add an extension on View
that makes use of the structure we simply wrote. This turns into our public API:
extension View {
/// Proposes a proportion of its obtained proposed measurement to `self`.
public func relativeProposed(width: Double = 1, top: Double = 1) -> some View {
RelativeSizeLayout(relativeWidth: width, relativeHeight: top) {
// Wrap content material view in a container to ensure the structure solely
// receives a single subview. As a result of views are lists!
VStack { // alternatively: `_UnaryViewAdaptor(self)`
self
}
}
}
}
Notes:
-
I made a decision to go along with a verbose title,
relativeProposed(width:top:)
, to make the semantics clear: we’re altering the proposed measurement for the subview, which received’t at all times end in a distinct precise measurement. Extra on this beneath. -
We’re wrapping the subview (
self
within the code above) in aVStack
. This might sound redundant, but it surely’s essential to ensure the structure solely receives a single factor in its subviews assortment. See Chris Eidhof’s SwiftUI Views are Lists for a proof.
Utilization
The structure code for a single chat bubble within the demo video above seems like this:
let alignment: Alignment = message.sender == .me ? .trailing : .main
chatBubble
.relativeProposed(width: 0.8)
.body(maxWidth: .infinity, alignment: alignment)
The outermost versatile body with maxWidth: .infinity
is answerable for positioning the chat bubble with main or trailing alignment, relying on who’s talking.
You may even add one other body that limits the width to a most, say 400 factors:
let alignment: Alignment = message.sender == .me ? .trailing : .main
chatBubble
.body(maxWidth: 400)
.relativeProposed(width: 0.8)
.body(maxWidth: .infinity, alignment: alignment)
Right here, our relative sizing modifier solely has an impact because the bubbles turn into narrower than 400 factors. In a wider window the width-limiting body takes priority. I like how composable that is!
80 % received’t at all times end in 80 %
In case you watch the debugging guides I’m drawing within the video above, you’ll discover that the relative sizing modifier by no means experiences a width better than 400, even when the window is vast sufficient:
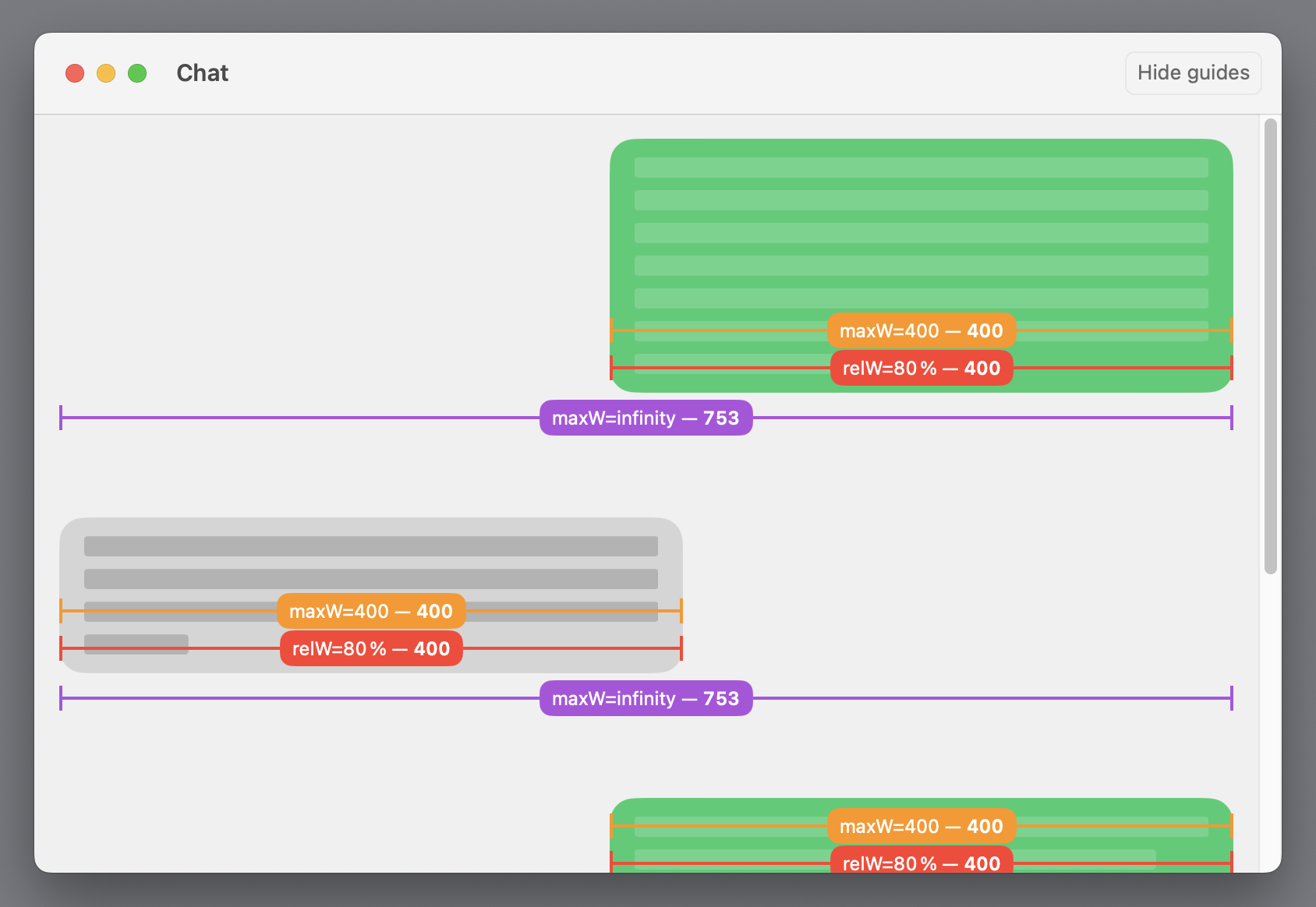
It is because our structure solely adjusts the proposed measurement for its subview however then accepts the subview’s precise measurement as its personal. Since SwiftUI views at all times select their very own measurement (which the mother or father can’t override), the subview is free to disregard our proposal. On this instance, the structure’s subview is the body(maxWidth: 400)
view, which units its personal width to the proposed width or 400, whichever is smaller.
Understanding the modifier’s conduct
Proposed measurement ≠ precise measurement
It’s vital to internalize that the modifier works on the idea of proposed sizes. This implies it relies on the cooperation of its subview to attain its objective: views that ignore their proposed measurement will probably be unaffected by our modifier. I don’t discover this significantly problematic as a result of SwiftUI’s total structure system works like this. In the end, SwiftUI views at all times decide their very own measurement, so you may’t write a modifier that “does the appropriate factor” (no matter that’s) for an arbitrary subview hierarchy.
nil
and infinity
I already talked about one other factor to pay attention to: if the mother or father of the relative sizing modifier proposes nil
or .infinity
, the modifier will move the proposal by unchanged. Once more, I don’t suppose that is significantly unhealthy, but it surely’s one thing to pay attention to.
Proposing nil
is SwiftUI’s approach of telling a view to turn into its excellent measurement (fixedSize
does this). Would you ever wish to inform a view to turn into, say, 50 % of its excellent width? I’m undecided. Perhaps it’d make sense for resizable photos and related views.
By the best way, you could possibly modify the structure to do one thing like this:
- If the proposal is
nil
or infinity, ahead it to the subview unchanged. - Take the reported measurement of the subview as the brand new foundation and apply the scaling components to that measurement (this nonetheless breaks down if the kid returns infinity).
- Now suggest the scaled measurement to the subview. The subview would possibly reply with a distinct precise measurement.
- Return this newest reported measurement as your personal measurement.
This means of sending a number of proposals to little one views is named probing. Plenty of built-in containers views do that too, e.g. VStack
and HStack
.
Nesting in different container views
The relative sizing modifier interacts in an fascinating approach with stack views and different containers that distribute the out there house amongst their kids. I assumed this was such an fascinating subject that I wrote a separate article about it: How the relative measurement modifier interacts with stack views.
The code
The whole code is out there in a Gist on GitHub.
Digression: Proportional sizing in early SwiftUI betas
The very first SwiftUI betas in 2019 did embody proportional sizing modifiers, however they have been taken out earlier than the ultimate launch. Chris Eidhof preserved a replica of SwiftUI’s “header file” from that point that exhibits their API, together with fairly prolonged documentation.
I don’t know why these modifiers didn’t survive the beta section. The discharge notes from 2019 don’t give a motive:
The
relativeWidth(_:)
,relativeHeight(_:)
, andrelativeSize(width:top:)
modifiers are deprecated. Use different modifiers likebody(minWidth:idealWidth:maxWidth:minHeight:idealHeight:maxHeight:alignment:)
as a substitute. (51494692)
I additionally don’t bear in mind how these modifiers labored. They in all probability had considerably related semantics to my resolution, however I can’t be certain. The doc feedback linked above sound simple (“Units the width of this view to the desired proportion of its mother or father’s width.”), however they don’t point out the intricacies of the structure algorithm (proposals and responses) in any respect.
[ad_2]