
[ad_1]
Round cells inside a set view
Reaching the purpose is comparatively straightforward, but when you do not know what is going on on within the background it should be tougher than you’d assume first. So let’s create a brand new venture add a storyboard with a UICollectionViewController
, drag a UIImageView
contained in the cell, resize it, add some constraints, set the cell identifier.
It ought to look one thing just like the picture above. Nothing particular only a easy UI for our instance utility. Now seek for some random picture, add it to the venture and let’s do some actual coding. First I am going to present you the little trick within the cell subclass.
class Cell: UICollectionViewCell {
@IBOutlet weak var imageView: UIImageView!
override var bounds: CGRect {
didSet {
layoutIfNeeded()
}
}
override func awakeFromNib() {
tremendous.awakeFromNib()
imageView.layer.masksToBounds = true
}
override func layoutSubviews() {
tremendous.layoutSubviews()
setCircularImageView()
}
func setCircularImageView() {
imageView.layer.cornerRadius = CGFloat(
roundf(Float(imageView.body.dimension.width / 2.0))
)
}
}
Are you able to see it? Sure, it is best to override the bounds property. As the subsequent step we’ve got to jot down the controller class with some fundamental information supply for the gathering view and with the right help for the rotation strategies. 🤓
class ViewController: UICollectionViewController {
override func collectionView(
_ collectionView: UICollectionView,
numberOfItemsInSection part: Int
) -> Int {
30
}
override func collectionView(
_ collectionView: UICollectionView,
cellForItemAt indexPath: IndexPath
) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(
withReuseIdentifier: "Cell",
for: indexPath
) as! Cell
cell.imageView.picture = UIImage(named: "Instance.jpg")
cell.imageView.backgroundColor = .lightGray
return cell
}
override func traitCollectionDidChange(
_ previousTraitCollection: UITraitCollection?
) {
tremendous.traitCollectionDidChange(previousTraitCollection)
guard
let previousTraitCollection = previousTraitCollection,
traitCollection.verticalSizeClass != previousTraitCollection.verticalSizeClass ||
traitCollection.horizontalSizeClass != previousTraitCollection.horizontalSizeClass
else {
return
}
collectionView?.collectionViewLayout.invalidateLayout()
collectionView?.reloadData()
}
override func viewWillTransition(
to dimension: CGSize,
with coordinator: UIViewControllerTransitionCoordinator
) {
tremendous.viewWillTransition(to: dimension, with: coordinator)
collectionView?.collectionViewLayout.invalidateLayout()
coordinator.animate(alongsideTransition: { context in
}, completion: { context in
collectionView?.collectionViewLayout.invalidateLayout()
collectionView?.visibleCells.forEach { cell in
guard let cell = cell as? Cell else {
return
}
cell.setCircularImageView()
}
})
}
}
extension ViewController: UICollectionViewDelegateFlowLayout {
func collectionView(
_ collectionView: UICollectionView,
format collectionViewLayout: UICollectionViewLayout,
sizeForItemAt indexPath: IndexPath
) -> CGSize {
.init(
width: collectionView.body.dimension.width/3.0 - 8,
peak: collectionView.body.dimension.width/3.0 - 8
)
}
}
In case you are conversant in assortment views, you may ask why am I doing this tutorial? It is so easy. It simply works, proper? No, really with out the overridden bounds property the instance would look one thing like this on the left facet. 😢
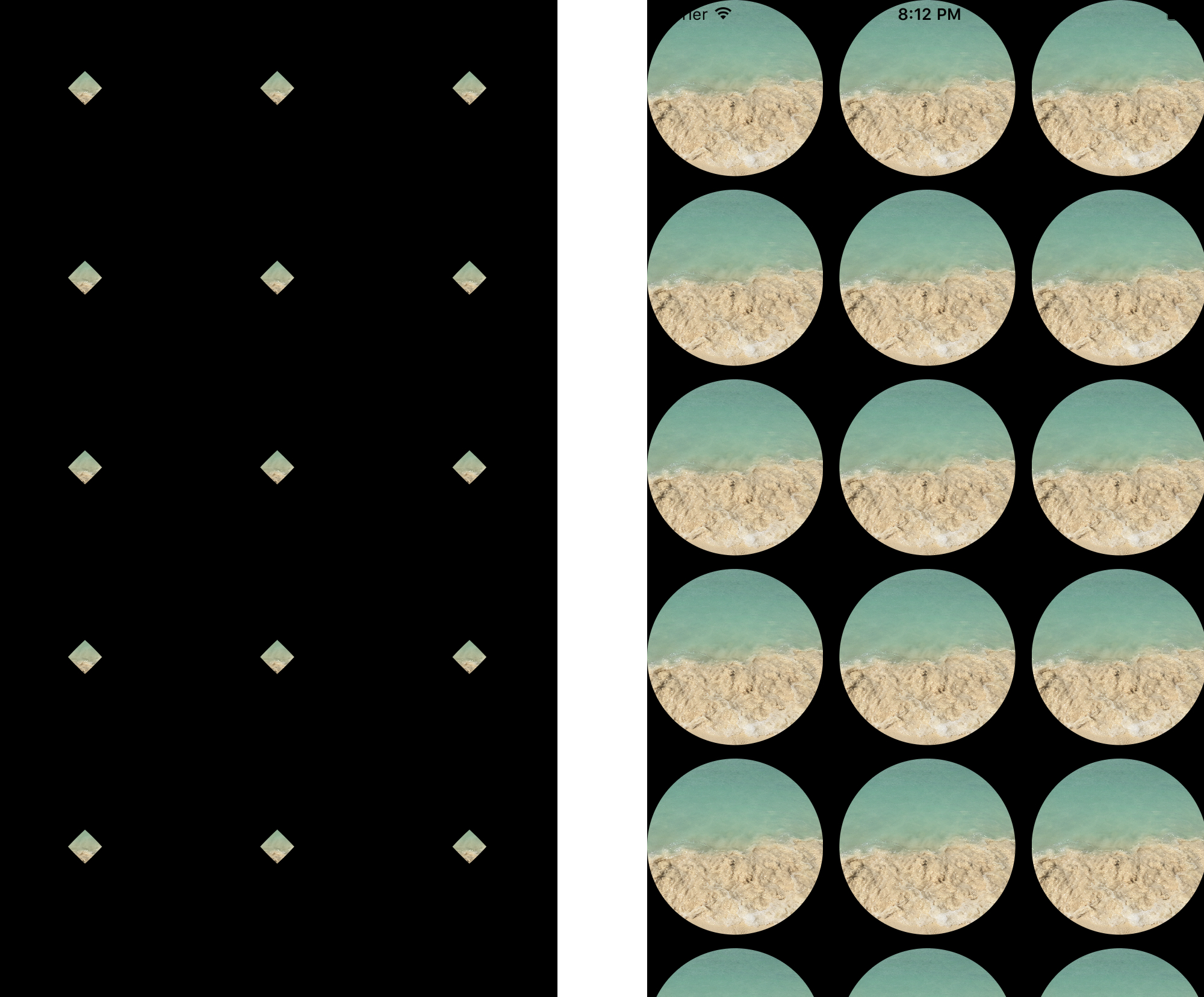
Humorous, huh? The picture on the appropriate facet is the precise outcome with the overridden bounds, that is the anticipated conduct. Scrolling and rotation goes to be actually unusual in case you do not override bounds and you do not reset the cornerRadius property for the seen views. You may ask: however why? 🤔
Layers, springs & struts and a few clarification
Apple nonetheless has “Springs & Struts” based mostly code within UIKit. Which means that body and sure calculations are taking place within the underlying system and the constraint system is attempting to work onerous as effectively to determine the right measures.
“Springs & Struts” must die!
Whereas there’s an init(body:)
technique, or a required init(coder:)
these format issues will suck as hell. I actually like Interface Builder, however till we can’t get a superb device to create nice consumer interfaces IB goes to be simply one other layer of doable bugs.
This subject will not even be there in case you create the cell from code solely utilizing auto format constraints or format anchors! It is as a result of IB creates the cell based mostly on the body you gave in when you designed your prototype. However in case you overlook init(body:)
and also you simply create a brand new UIImageView
occasion and let auto format do the onerous work, the format system will resolve all the pieces else. Verify this.
class Cell: UICollectionViewCell {
weak var imageView: UIImageView!
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been carried out")
}
override init(body: CGRect) {
tremendous.init(body: body)
translatesAutoresizingMaskIntoConstraints = false
let imageView = UIImageView()
imageView.translatesAutoresizingMaskIntoConstraints = false
addSubview(imageView)
imageView = imageView
imageView.topAnchor.constraint(equalTo: topAnchor)
imageView.bottomAnchor.constraint(equalTo: bottomAnchor)
imageView.leadingAnchor.constraint(equalTo: leadingAnchor)
imageView.trailingAnchor.constraint(equalTo: trailingAnchor)
}
override func layoutSubviews() {
tremendous.layoutSubviews()
imageView.layer.masksToBounds = true
imageView.layer.cornerRadius = CGFloat(
roundf(Float(imageView.body.dimension.width/2.0))
)
}
}
Clearly it’s important to write extra code, register your cell class manually contained in the controller class and also you additionally must override the layoutSubviews technique contained in the cell, nevertheless it’ll work as it’s anticipated. 🙄
collectionView?.register(Cell.self, forCellWithReuseIdentifier: "Cell")
Anyway, after you register the programmatically created cell you may have a pleasant method of displaying round photos. Utilizing this system is kind of difficult, nevertheless it undoubtedly works in each case. You possibly can obtain the instance from The.Swift.Dev. tutorials on GitHub.
[ad_2]