
[ad_1]
Python is an indispensable instrument for knowledge science professionals, taking part in a pivotal position in knowledge evaluation, machine studying, and scientific computing. Whether or not you’re a novice or an skilled practitioner, enhancing your Python programming expertise is an ongoing journey. This text is your gateway to 14 thrilling Python venture concepts, every fastidiously crafted to cater to the wants of knowledge science lovers. These initiatives provide a novel alternative to not solely enhance your Python expertise but additionally create sensible functions that may be utilized in your data-driven endeavors.
So, let’s start our Python venture journey!
High 14 Mini Python Initiatives
Calculator
A beginner-friendly Python venture concept is to create a fundamental calculator. This program performs basic mathematical operations, corresponding to addition, subtraction, multiplication, and division. You possibly can additional improve it by including options like reminiscence features or historical past monitoring. Constructing a calculator is a good way to observe Python’s core syntax and mathematical operations.
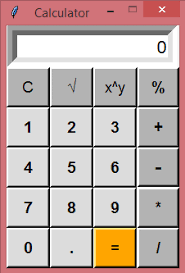
Python Code
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Can not divide by zero"
return x / y
whereas True:
print("Choices:")
print("Enter 'add' for addition")
print("Enter 'subtract' for subtraction")
print("Enter 'multiply' for multiplication")
print("Enter 'divide' for division")
print("Enter 'exit' to finish this system")
user_input = enter(": ")
if user_input == "exit":
break
if user_input in ("add", "subtract", "multiply", "divide"):
num1 = float(enter("Enter first quantity: "))
num2 = float(enter("Enter second quantity: "))
if user_input == "add":
print("Outcome: ", add(num1, num2))
elif user_input == "subtract":
print("Outcome: ", subtract(num1, num2))
elif user_input == "multiply":
print("Outcome: ", multiply(num1, num2))
elif user_input == "divide":
print("Outcome: ", divide(num1, num2))
else:
print("Invalid enter")
To-Do Checklist
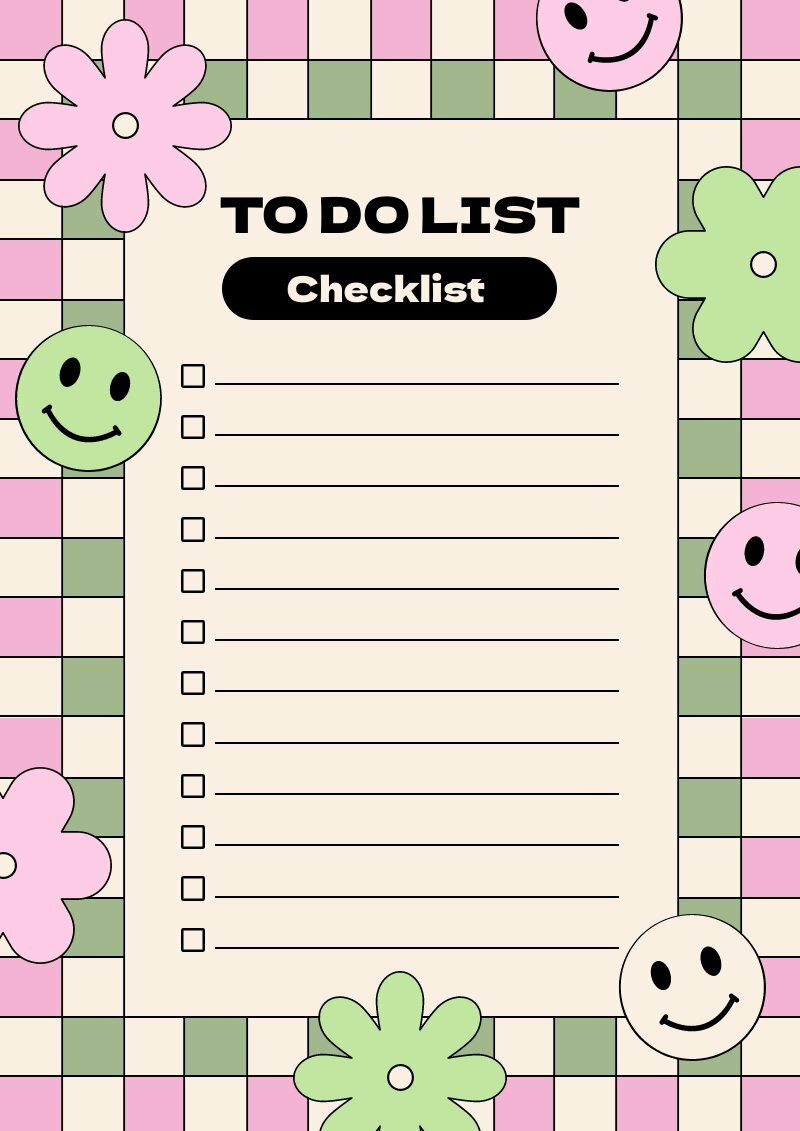
A to-do checklist software is a useful instrument for organizing duties. Create a fundamental Python program that enables customers so as to add, delete, and examine duties. This straightforward venture helps learners perceive knowledge storage and manipulation. As you progress, you possibly can improve it with options like due dates, priorities, and extra, making it a precious instrument for private process administration.
Python Code
# Outline an empty checklist to retailer duties
duties = []
def add_task(process):
duties.append(process)
print("Job added:", process)
def delete_task(process):
if process in duties:
duties.take away(process)
print("Job deleted:", process)
else:
print("Job not discovered")
def view_tasks():
if not duties:
print("No duties within the checklist")
else:
print("Duties:")
for index, process in enumerate(duties, begin=1):
print(f"{index}. {process}")
whereas True:
print("Choices:")
print("Enter 'add' so as to add a process")
print("Enter 'delete' to delete a process")
print("Enter 'view' to view duties")
print("Enter 'exit' to finish this system")
user_input = enter(": ")
if user_input == "exit":
break
elif user_input == "add":
process = enter("Enter a process: ")
add_task(process)
elif user_input == "delete":
process = enter("Enter the duty to delete: ")
delete_task(process)
elif user_input == "view":
view_tasks()
else:
print("Invalid enter")
Guess the Quantity
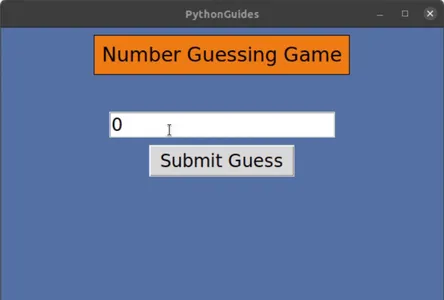
“Guess the Quantity” is a traditional Python venture the place the pc selects a random quantity, and the participant’s process is to guess it. Gamers can enter their guesses, and this system gives hints, progressively difficult the sport. It’s a fascinating and academic venture that enhances your understanding of random quantity technology and person interactions.
Python Code
import random
# Generate a random quantity between 1 and 100
secret_number = random.randint(1, 100)
makes an attempt = 0
print("Welcome to the Guess the Quantity sport!")
print("I am considering of a quantity between 1 and 100.")
whereas True:
strive:
guess = int(enter("Your guess: "))
makes an attempt += 1
if guess < secret_number:
print("Strive the next quantity.")
elif guess > secret_number:
print("Strive a decrease quantity.")
else:
print(f"Congratulations! You've got guessed the quantity in {makes an attempt} makes an attempt.")
break
besides ValueError:
print("Invalid enter. Please enter a quantity between 1 and 100.")
print("Thanks for taking part in!")
Fundamental Internet Scraper
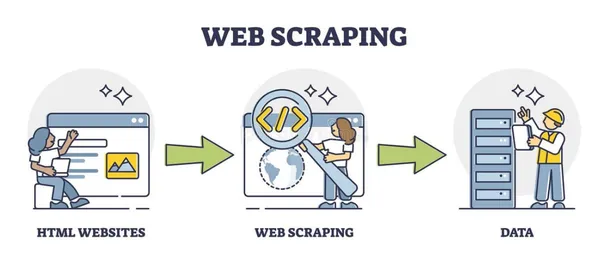
Create a fundamental net scraper in Python to extract knowledge from web sites. This venture helps you perceive net scraping ideas and HTTP requests. Begin by fetching info from a easy webpage and regularly progress to extra complicated scraping duties. This venture gives precious insights into knowledge acquisition and manipulation utilizing Python.
Python Code
import requests
from bs4 import BeautifulSoup
# URL of the webpage you need to scrape
url = "https://instance.com"
# Ship an HTTP GET request to the URL
response = requests.get(url)
# Examine if the request was profitable (standing code 200)
if response.status_code == 200:
# Parse the HTML content material of the web page utilizing BeautifulSoup
soup = BeautifulSoup(response.textual content, 'html.parser')
# Extract knowledge from the webpage (for instance, scraping all of the hyperlinks)
hyperlinks = []
for hyperlink in soup.find_all('a'):
hyperlinks.append(hyperlink.get('href'))
# Print the scraped knowledge (on this case, the hyperlinks)
for hyperlink in hyperlinks:
print(hyperlink)
else:
print("Didn't fetch the webpage. Standing code:", response.status_code)
Phrase Counter
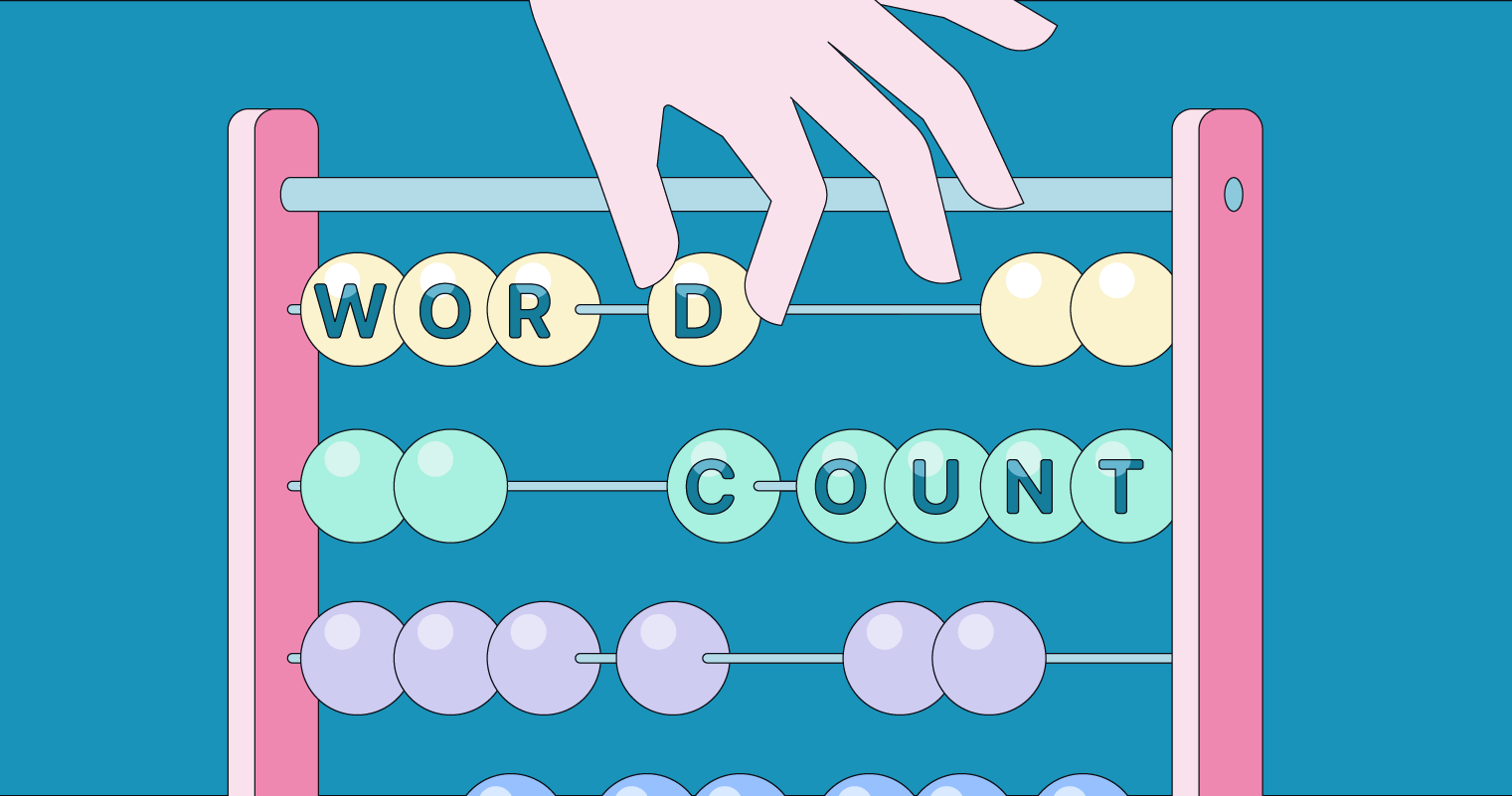
A Phrase Counter is a straightforward Python venture the place you create a program to rely the variety of phrases in a textual content. It’s a superb train for learners, serving to them find out about string manipulation and fundamental textual content evaluation. You possibly can later increase it to rely characters, sentences, or paragraphs for a extra versatile instrument.
Python Code
def count_words(textual content):
# Break up the textual content into phrases utilizing whitespace as a delimiter
phrases = textual content.cut up()
return len(phrases)
# Get enter textual content from the person
textual content = enter("Enter some textual content: ")
# Name the count_words perform to rely the phrases
word_count = count_words(textual content)
# Show the phrase rely
print(f"Phrase rely: {word_count}")
Hangman Recreation
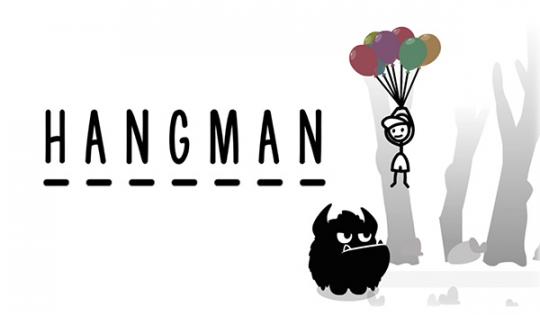
The Hangman Recreation is a traditional word-guessing pastime delivered to life in Python. On this participating venture, gamers try and guess a phrase letter by letter. This system can embody various phrase choices and even a scoring system, making it an entertaining and academic venture for learners.
Python Code
import random
# Checklist of phrases to select from
phrases = ["python", "hangman", "computer", "programming", "challenge"]
# Perform to decide on a random phrase
def choose_word():
return random.alternative(phrases)
# Perform to show the present state of the phrase with blanks
def display_word(phrase, guessed_letters):
show = ""
for letter in phrase:
if letter in guessed_letters:
show += letter
else:
show += "_"
return show
# Perform to play Hangman
def play_hangman():
word_to_guess = choose_word()
guessed_letters = []
makes an attempt = 6
print("Welcome to Hangman!")
whereas makes an attempt > 0:
print("nWord: " + display_word(word_to_guess, guessed_letters))
print("Makes an attempt left:", makes an attempt)
guess = enter("Guess a letter: ").decrease()
if len(guess) == 1 and guess.isalpha():
if guess in guessed_letters:
print("You've got already guessed that letter.")
elif guess in word_to_guess:
print("Good guess!")
guessed_letters.append(guess)
if set(word_to_guess).issubset(set(guessed_letters)):
print("Congratulations! You've got guessed the phrase:", word_to_guess)
break
else:
print("Incorrect guess!")
guessed_letters.append(guess)
makes an attempt -= 1
else:
print("Invalid enter. Please enter a single letter.")
if makes an attempt == 0:
print("You ran out of makes an attempt. The phrase was:", word_to_guess)
# Begin the sport
play_hangman()
Easy Alarm Clock
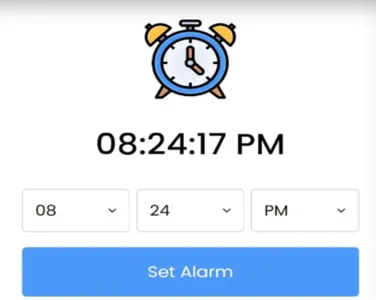
A Easy Alarm Clock venture includes making a Python software that enables customers to set alarms, with options like snooze and cease choices. It’s a superb venture for learners to delve into time and date dealing with in Python. This hands-on expertise can lay the muse for extra complicated functions and assist customers achieve sensible programming expertise.
Python Code
import time
import winsound
# Perform to set an alarm
def set_alarm():
alarm_time = enter("Enter the alarm time (HH:MM): ")
whereas True:
current_time = time.strftime("%H:%M")
if current_time == alarm_time:
print("Get up!")
winsound.Beep(1000, 1000) # Beep for 1 second
break
# Perform to snooze the alarm
def snooze_alarm():
snooze_time = 5 # Snooze for five minutes
alarm_time = time.time() + snooze_time * 60
whereas time.time() < alarm_time:
go
print("Snooze time is over. Get up!")
winsound.Beep(1000, 1000) # Beep for 1 second
# Perform to cease the alarm
def stop_alarm():
print("Alarm stopped")
# Primary loop for the alarm clock
whereas True:
print("Choices:")
print("Enter '1' to set an alarm")
print("Enter '2' to snooze the alarm")
print("Enter '3' to cease the alarm")
print("Enter '4' to exit")
alternative = enter(": ")
if alternative == '1':
set_alarm()
elif alternative == '2':
snooze_alarm()
elif alternative == '3':
stop_alarm()
elif alternative == '4':
break
else:
print("Invalid enter. Please enter a sound possibility.")
Cube Curler
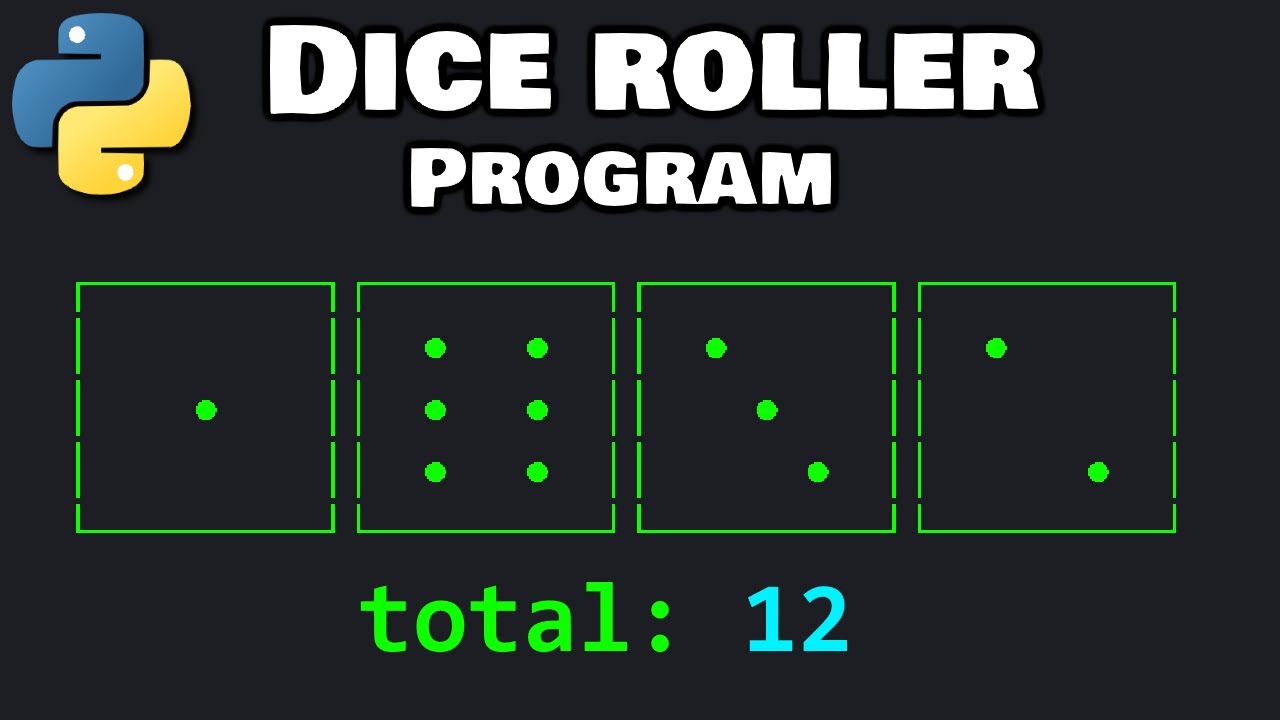
The Cube Curler venture is a enjoyable Python endeavor that simulates rolling cube. Random quantity technology permits customers to roll numerous kinds of cube, from customary six-sided ones to extra unique varieties. It’s a easy but entertaining strategy to delve into Python’s randomization capabilities and create an interactive dice-rolling expertise.
Python Code
import random
def roll_dice(sides):
return random.randint(1, sides)
whereas True:
print("Choices:")
print("Enter 'roll' to roll a cube")
print("Enter 'exit' to finish this system")
user_input = enter(": ")
if user_input == "exit":
break
if user_input == "roll":
sides = int(enter("Enter the variety of sides on the cube: "))
end result = roll_dice(sides)
print(f"You rolled a {sides}-sided cube and bought: {end result}")
else:
print("Invalid enter. Please enter a sound possibility.")
Mad Libs Generator
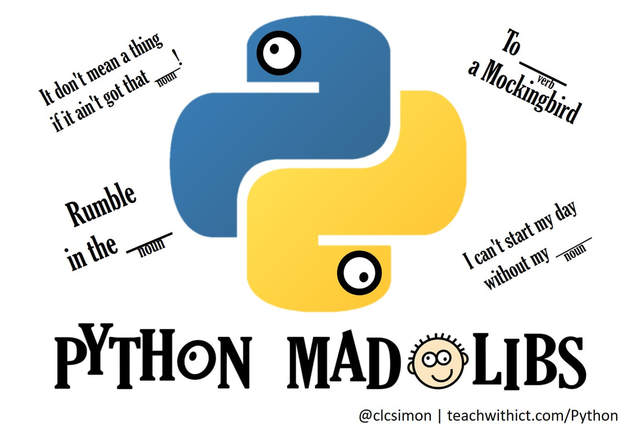
The Mad Libs Generator is a artistic and entertaining Python venture. It prompts customers to enter numerous phrase sorts (nouns, verbs, adjectives) after which generates amusing tales utilizing their phrases. This venture is enjoyable and a very good train in string manipulation and person interplay. It’s a playful strategy to discover the world of Python programming.
Python Code
# Outline a Mad Libs story template
mad_libs_template = "As soon as upon a time, in a {adjective} {place}, there lived a {animal}. It was a {adjective} and {coloration} {animal}. At some point, the {animal} {verb} to the {place} and met a {adjective} {particular person}. They turned quick associates and went on an journey to {verb} the {noun}."
# Create a dictionary to retailer person inputs
user_inputs = {}
# Perform to get person inputs
def get_user_input(immediate):
worth = enter(immediate + ": ")
return worth
# Change placeholders within the story template with person inputs
def generate_mad_libs_story(template, inputs):
story = template.format(**inputs)
return story
# Get person inputs for the Mad Libs
for placeholder in ["adjective", "place", "animal", "color", "verb", "person", "noun"]:
user_inputs[placeholder] = get_user_input(f"Enter a {placeholder}")
# Generate the Mad Libs story
mad_libs_story = generate_mad_libs_story(mad_libs_template, user_inputs)
# Show the generated story
print("nHere's your Mad Libs story:")
print(mad_libs_story)
Password Generator
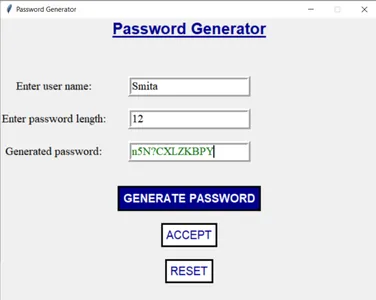
The Password Generator venture includes making a Python program that generates robust, safe passwords based mostly on person preferences. Customers can specify parameters corresponding to password size and character sorts, and this system will output a sturdy password, enhancing cybersecurity for on-line accounts and private knowledge.
Python Code
import random
import string
def generate_password(size, use_uppercase, use_digits, use_special_chars):
characters = string.ascii_lowercase
if use_uppercase:
characters += string.ascii_uppercase
if use_digits:
characters += string.digits
if use_special_chars:
characters += string.punctuation
if len(characters) == 0:
print("Error: No character sorts chosen. Please allow not less than one possibility.")
return None
password = ''.be a part of(random.alternative(characters) for _ in vary(size))
return password
def primary():
print("Password Generator")
whereas True:
size = int(enter("Enter the password size: "))
use_uppercase = enter("Embrace uppercase letters? (sure/no): ").decrease() == "sure"
use_digits = enter("Embrace digits? (sure/no): ").decrease() == "sure"
use_special_chars = enter("Embrace particular characters? (sure/no): ").decrease() == "sure"
password = generate_password(size, use_uppercase, use_digits, use_special_chars)
if password:
print("Generated Password:", password)
one other = enter("Generate one other password? (sure/no): ").decrease()
if one other != "sure":
break
if __name__ == "__main__":
primary()
Fundamental Textual content Editor
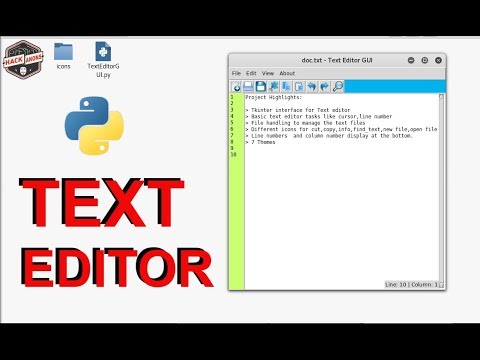
A fundamental textual content editor is a straightforward software program software for creating and enhancing plain textual content paperwork. It gives important features like typing, copying, chopping, pasting, and fundamental formatting. Whereas missing superior options in phrase processors, it’s light-weight, fast to make use of, and appropriate for duties like note-taking or writing code. Standard examples embody Notepad (Home windows) and TextEdit (macOS), offering a user-friendly atmosphere for minimalistic textual content manipulation.
Python Code
import tkinter as tk
from tkinter import filedialog
def new_file():
textual content.delete(1.0, tk.END)
def open_file():
file_path = filedialog.askopenfilename(filetypes=[("Text Files", "*.txt")])
if file_path:
with open(file_path, "r") as file:
textual content.delete(1.0, tk.END)
textual content.insert(tk.END, file.learn())
def save_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text Files", "*.txt")])
if file_path:
with open(file_path, "w") as file:
file.write(textual content.get(1.0, tk.END))
# Create the principle window
root = tk.Tk()
root.title("Fundamental Textual content Editor")
# Create a menu
menu = tk.Menu(root)
root.config(menu=menu)
file_menu = tk.Menu(menu)
menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="New", command=new_file)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.stop)
# Create a textual content space
textual content = tk.Textual content(root, wrap=tk.WORD)
textual content.pack(increase=True, fill="each")
# Begin the GUI primary loop
root.mainloop()
Mini Climate App
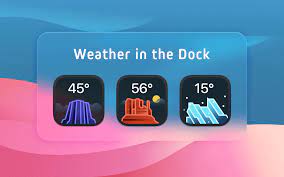
The Mini Climate App is a compact instrument for fast climate updates. Customers can entry present situations and forecasts in a concise interface. It gives important info like temperature, precipitation, and wind pace, guaranteeing customers keep knowledgeable on the go. This light-weight app is ideal for these searching for instant climate insights with out the litter.
Python Code
import requests
def get_weather(metropolis, api_key):
base_url = "https://api.openweathermap.org/knowledge/2.5/climate"
params = {
"q": metropolis,
"appid": api_key,
"models": "metric" # Change to "imperial" for Fahrenheit
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
weather_data = response.json()
temperature = weather_data["main"]["temp"]
description = weather_data["weather"][0]["description"]
humidity = weather_data["main"]["humidity"]
wind_speed = weather_data["wind"]["speed"]
print(f"Climate in {metropolis}:")
print(f"Temperature: {temperature}°C")
print(f"Description: {description}")
print(f"Humidity: {humidity}%")
print(f"Wind Pace: {wind_speed} m/s")
else:
print("Didn't fetch climate knowledge. Please test your metropolis identify and API key.")
def primary():
print("Mini Climate App")
metropolis = enter("Enter a metropolis: ")
api_key = enter("Enter your OpenWeatherMap API key: ")
get_weather(metropolis, api_key)
if __name__ == "__main__":
primary()
Fundamental Paint Software
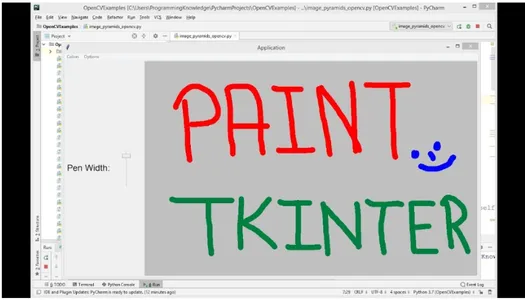
A fundamental paint software is a user-friendly software program that enables customers to create and edit digital photos utilizing numerous instruments like brushes, colours, and shapes. It’s best for easy graphic design and digital artwork, providing important drawing, coloring, and fundamental picture manipulation options. Whereas missing superior options, it’s a nice place to begin for novice artists and designers.
Python Code
import tkinter as tk
from tkinter import colorchooser
def start_paint(occasion):
world prev_x, prev_y
prev_x, prev_y = occasion.x, occasion.y
def paint(occasion):
x, y = occasion.x, occasion.y
canvas.create_line((prev_x, prev_y, x, y), fill=current_color, width=brush_size, capstyle=tk.ROUND, easy=tk.TRUE)
prev_x, prev_y = x, y
def choose_color():
world current_color
coloration = colorchooser.askcolor()[1]
if coloration:
current_color = coloration
def change_brush_size(new_size):
world brush_size
brush_size = new_size
root = tk.Tk()
root.title("Fundamental Paint Software")
current_color = "black"
brush_size = 2
prev_x, prev_y = None, None
canvas = tk.Canvas(root, bg="white")
canvas.pack(fill=tk.BOTH, increase=True)
canvas.bind("<Button-1>", start_paint)
canvas.bind("<B1-Movement>", paint)
menu = tk.Menu(root)
root.config(menu=menu)
options_menu = tk.Menu(menu, tearoff=0)
menu.add_cascade(label="Choices", menu=options_menu)
options_menu.add_command(label="Select Coloration", command=choose_color)
options_menu.add_command(label="Brush Dimension (1)", command=lambda: change_brush_size(1))
options_menu.add_command(label="Brush Dimension (3)", command=lambda: change_brush_size(3))
options_menu.add_command(label="Brush Dimension (5)", command=lambda: change_brush_size(5))
root.mainloop()
Fundamental Chat Software
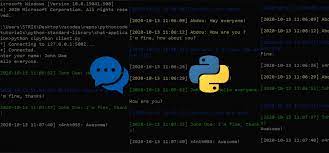
A fundamental chat software allows real-time textual content communication between customers. Customers can ship and obtain messages, creating one-on-one or group conversations. Options usually embody message typing indicators, learn receipts, and person profiles. These apps are standard for private and enterprise communication, fostering instantaneous, handy, and infrequently asynchronous exchanges of data and concepts.
Server Python Code
import socket
import threading
# Server configuration
HOST = '0.0.0.0' # Pay attention on all obtainable community interfaces
PORT = 12345
# Create a socket for the server
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((HOST, PORT))
server_socket.pay attention()
purchasers = []
def handle_client(client_socket):
whereas True:
strive:
message = client_socket.recv(1024).decode("utf-8")
if not message:
remove_client(client_socket)
else:
broadcast(message, client_socket)
besides Exception as e:
print(f"An error occurred: {str(e)}")
remove_client(client_socket)
break
def remove_client(client_socket):
if client_socket in purchasers:
purchasers.take away(client_socket)
def broadcast(message, client_socket):
for consumer in purchasers:
if consumer != client_socket:
strive:
consumer.ship(message.encode("utf-8"))
besides Exception as e:
remove_client(consumer)
print(f"An error occurred: {str(e)}")
def primary():
print("Chat server is listening on port", PORT)
whereas True:
client_socket, addr = server_socket.settle for()
purchasers.append(client_socket)
client_handler = threading.Thread(goal=handle_client, args=(client_socket,))
client_handler.begin()
if __name__ == "__main__":
primary()
Shopper Python Code
import socket
import threading
# Shopper configuration
HOST = '127.0.0.1' # Server's IP deal with
PORT = 12345
# Create a socket for the consumer
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.join((HOST, PORT))
def receive_messages():
whereas True:
strive:
message = client_socket.recv(1024).decode("utf-8")
print(message)
besides Exception as e:
print(f"An error occurred: {str(e)}")
client_socket.shut()
break
def send_messages():
whereas True:
message = enter()
client_socket.ship(message.encode("utf-8"))
def primary():
print("Linked to the chat server.")
receive_thread = threading.Thread(goal=receive_messages)
receive_thread.begin()
send_thread = threading.Thread(goal=send_messages)
send_thread.begin()
if __name__ == "__main__":
primary()
Significance of Python in Knowledge Science
Python holds a paramount place within the realm of knowledge science as a result of its versatility, effectivity, and an intensive ecosystem of libraries and instruments tailor-made for knowledge evaluation. Its significance lies in a number of key points:
- Ease of Studying and Use: Python’s easy and readable syntax makes it accessible for each learners and specialists, expediting the educational curve for knowledge science practitioners.
- Ample Libraries: Python boasts highly effective libraries like NumPy for numerical operations, Pandas for knowledge manipulation, Matplotlib and Seaborn for visualization, and Scikit-Be taught for machine studying. This library-rich atmosphere streamlines complicated knowledge evaluation duties.
- Neighborhood Assist: The huge Python group constantly develops and maintains knowledge science libraries. This ensures that instruments are up-to-date, dependable, and backed by a supportive person group.
- Machine Studying and AI: Python serves as a hub for machine studying and AI growth, providing libraries like TensorFlow, Keras, and PyTorch. These instruments simplify the creation and deployment of predictive fashions.
- Knowledge Visualization: Python allows the creation of compelling knowledge visualizations, enhancing the understanding and communication of knowledge insights.
- Integration: Python seamlessly integrates with databases, net frameworks, and massive knowledge applied sciences, making it a superb alternative for end-to-end knowledge science pipelines.
Additionally Learn: High 10 Makes use of of Python within the Actual World
Conclusion
These 14 small Python initiatives provide a superb place to begin for learners to boost their coding expertise. They supply sensible insights into Python’s core ideas. As you embark in your programming journey, keep in mind that observe is essential to mastery.
In the event you’re ready to maneuver ahead, take into account signing up for Analytics Vidhya’s Python course. You’ll achieve in-depth schooling, sensible expertise, and the prospect to work on precise knowledge science initiatives. This course is even for learners who’ve a coding or Knowledge Science background. Benefit from the chance to additional your profession and develop your Python expertise. Be a part of our Python course as we speak!
Associated
[ad_2]